How to enable Route tracing - Angular 2+
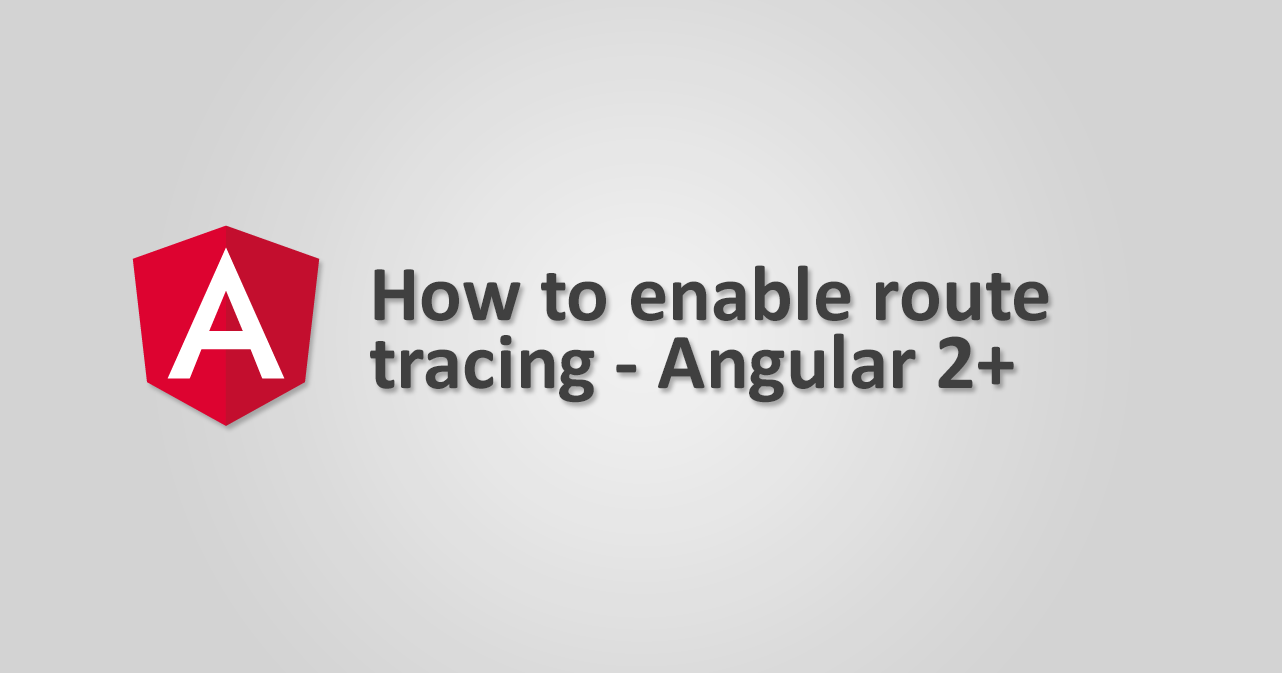
Debugging an Angular app route configuration may eat up your costly time. As it may get tricky to enable route logs. Many Angular beginners frequently ask me ways to debug issues with angular routing. In this post I will explain two simple techniques using which you can detect, trace and debug your route changes in Angular.
RouterModule enableTracing
Angular has built in feature to log all route change events. However route log tracing is disabled by default, since it may annoy developers. Set the enableTracing
flag in the root route configuration, to enable route logs.
|
|
Note: Generally app-routing.module.ts or app.module.ts file inject root route. But can vary from project to project.
Save it and let angular compile your project again. Tada, you can see group of logs on the console on each route change.
The above method logs all the router event changes to the console. However, you might not always want to see all router logs. Several times you want some specific router event logs. Which calls us for the second way to enable angular router trace.
Router events
Router class provides several useful fields and functions. Out of all in this post we will talk about one special member of Router class. Router.events
provides way to access all router events. You can subscribe to the field to get notified on any route change events.
|
|
This too prints the same output on console. But, our intention was to filter specific router event. You can apply filter to the Router.events
stream using rxjs filter operator.
E.g. Following code logs only NavigationStart
events.
|
|
Note: Since you are subscribing to an observable (
Router.events
) you must unsubscribe to it after the use. Possibly add unsubscription logic when component gets destroyed (ngOnDestroy
). Otherwise it leads to memory leaks.
Happy coding 👨💻