How to Integrate Google Maps in Angular App
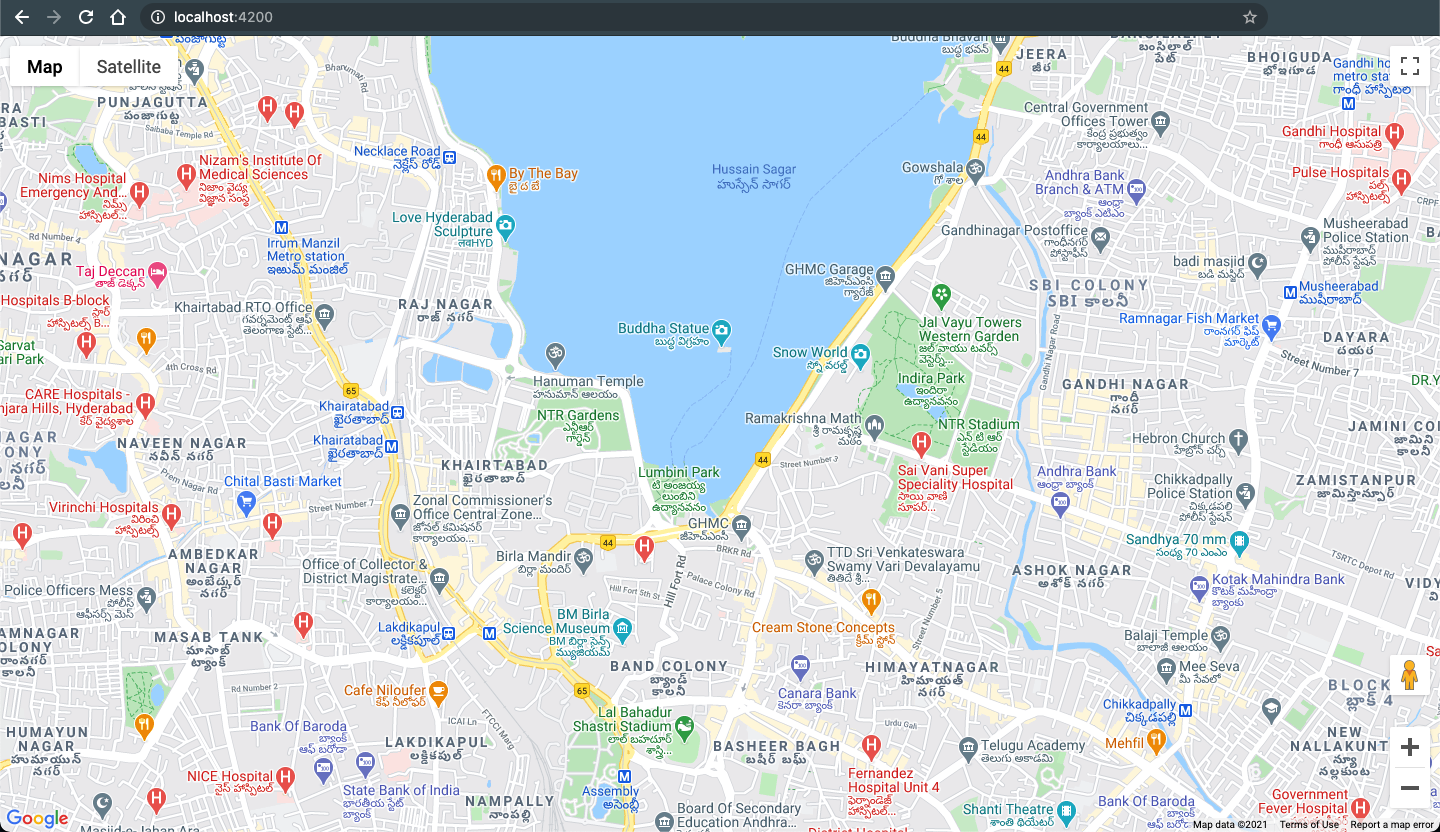
You might have came across several applications with maps. From open source to subscription based, there are several maps options available over internet. In this tutorial series, I will explain you how easily you can integrate google maps in your angular application.
Google Maps is a subscription based maps solution. You must create a billing account to integrate google maps in your app.
Please read the Google Maps Platform starter guide. It will help you to get started with a new google billing account and API key, which you will require later in this project.
Create an angular app
Let us create a new angular app. We will use this app for this tutorial series. Open terminal and hit following command.
|
|
Note: This is optional step if you want to integrate google maps in your existing angular app.
Install types
Installing types support for google maps helps immensely with intellisense in most of the modern code editors and IDE.
Open your angular project root directory and install following package.
|
|
Let the typescript server know about the new typings. Open tsconfig.json
and add a new item to "compilerOptions.types"
array.
Ensure your tsconfig.json
file looks similar to following.
|
|
One final step to ensure your typescript server knows about the typings. Add following line at the beginning of main.ts
file.
|
|
Load Google Maps JS API
Before continuing to this step ensure you have created a new google billing account. If not, at least you have generated a new API key.
There are different ways to load google maps JS API. However, for the sake of simplicity we will go with the easiest solution.
We will load our google maps JS API in index.html
file. Add following line to your index.html
file. Ensure you replace API_KEY
with your google maps API key.
|
|
Google Maps API
Google Maps provides google.maps.Map
class. Using this we can create a new instance of map and render to the DOM. The class excepts two parameters.
-
Instance of DOM element where we want to render our map.
-
Object of type
google.maps.MapOptions
that defines extra properties used to customize the map. You must provide at least two params asMapOptions
i.e.center
andzoom
.center
Object specifying initial latitude and longitude for the map to focus.zoom
An integer that specifies the initial resolution for the map. You can specify a zoom level between 0-20.
The below list shows approximate level of detail you can expect to see at each zoom level.
- 1: World
- 5: Landmass/continent
- 10: City
- 15: Streets
- 20: Buildings
Integrate in your app
Keeping the legacy intact, let us start with a hello world example. Run below command to generate hello-world
component.
|
|
Create DOM element to render map
Add a new div
to our hello-world.component.html
. This is the place where our map gets rendered.
|
|
Note: We will use #googleMap
as a reference point to get the instance of div
element later.
For now let us adjust the height and width of the map. Open hello-world.component.scss
and add below lines.
|
|
Render map to DOM
To render a map we will use below MapOptions
object.
|
|
You can get the instance of DOM element using @ViewChild
in angular.
|
|
Let’s bring it all together.
|
|
Run application
Happy Coding 👨💻
References